Okay, imagine Git as a helpful friend who keeps track of changes in your files when you’re working on a project. Git hooks are like a little assistant that helps Git do extra things automatically whenever you make changes or do specific actions.
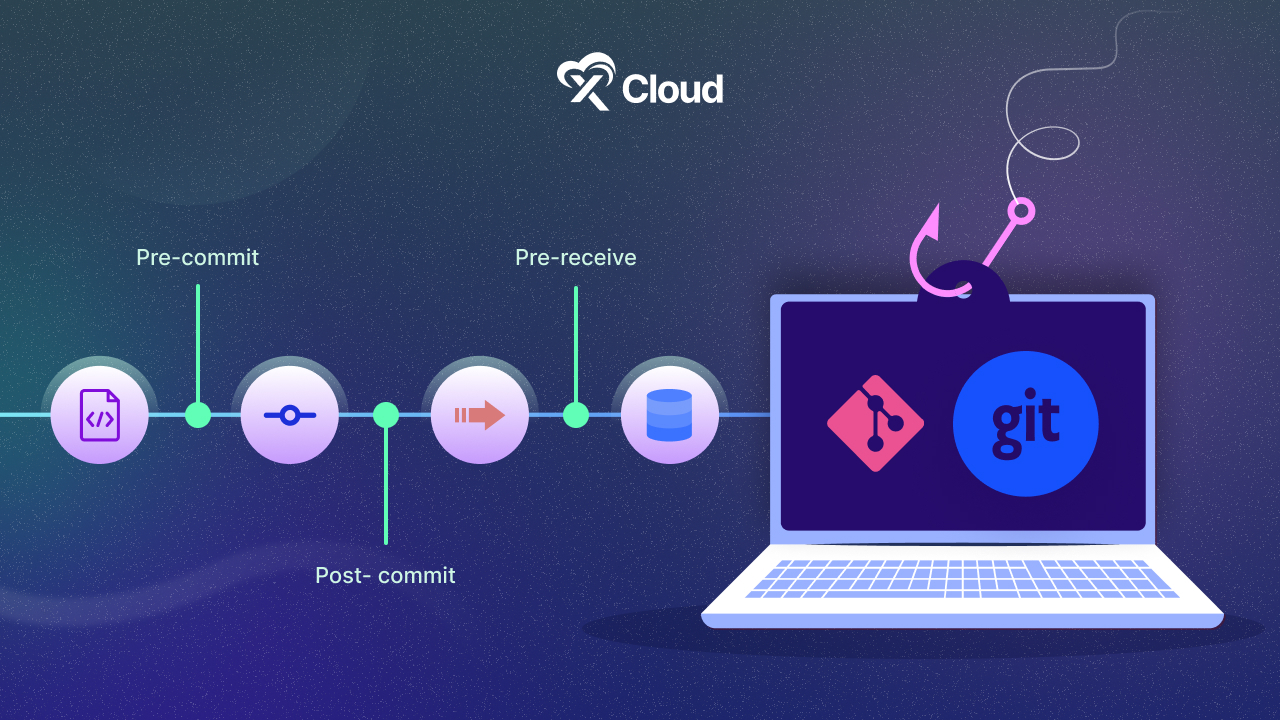
Here’s a super simple example: Let’s say you want to make sure your code passes some tests before you can add it to your project. You can use a Git hook to automatically run those tests whenever you try to “commit” your changes (committing is like saving your work in Git).
So, you create a file called pre-commit in a special folder where Git looks for hooks. Inside this file, you write a command that runs your tests. Now, every time you try to commit your changes, Git will first run those tests. If the tests pass, you can commit your changes. If they don’t, Git will stop you and ask you to fix things before you can commit.
Understanding The Basics of Git
To truly use Git well, it’s important to understand its basics. Git, made by Linus Torvalds in 2005, is the gold standard for managing code. It’s fast, handles big projects well, and lets many people work on code together easily. Let’s look at the main ideas and key commands:
💡Main Ideas
⚙️ (Repo): It’s like a folder that holds all your project files and their history. Everything Git knows about your project is stored in a special folder called .git.
⚙️ Local Repository: This is your project on your computer. It has all your files, the history of changes you made, and any different versions (branches) you’re working on.
⚙️ Remote Repository: This is your project stored on a server like GitHub. It’s where everyone on your team can see and share changes.
⚙️ Commit: A commit is like a snapshot of your project at a certain time. It remembers all the changes you’ve made, who made them, and when.
⚙️ Branch: Think of branches as different versions of your project. You can work on one without messing up the others. By default, the main branch is usually called “master.”
⚙️ HEAD: It’s like a pointer to the latest commit in the branch you’re working on. It’s where your changes start from.
⚙️Remote Tracking Branch: This is like a bookmark to where the branches are on the remote server. It helps keep track of changes made by others.
📣 Some key commands of git
git init | Set up a new Git repository in your current folder. |
git clone <repository-url> | Copies a repository from a remote server (like GitHub) to your computer |
git add <file> or git add | Adds changes you made to files so Git can track them. |
git commit -m “Commit message” | Saves your changes to the repository with a message explaining what you did. |
git status | Shows what changes you’ve made that Git knows about |
git log | Lists all the commits you’ve made, who made them, and when |
git push <remote-name> <branch-name> | Sends your commits to the remote repository. |
git pull <remote-name> <branch-name> | Gets changes from the remote repository and merges them into your current branch |
git branch | Shows all the branches in your repository or lets you make a new one. |
git checkout <branch-name> or git checkout <commit-id> <file> | Switches between branches or restores files from an earlier commit. |
These basics are really important for working on code with others. As you get more used to Git, you can learn more advanced stuff like how to manage branches, combine changes from different branches, and work together smoothly. Git is great for teams of all sizes because it’s flexible and works well, making it easy for everyone to manage their code projects.
Types Of Git Hooks
In Git, hooks are classified into two types based on their scope within the repository:
- Client-Side Hooks (Local Hooks):
- Also known as local hooks, these are specific to individual developers’ repositories.
- Located in the .git/hooks directory of each local repository.
- Trigger actions on a developer’s local machine before or after specific Git events, like committing or pushing changes.
- Help maintain personal development standards and code quality.
- Server-Side Hooks:
- Applied to the remote repository, serving as the central collaboration hub for a team.
- Reside on the server hosting the remote repository.
- Activate certain events on the remote repository, such as receiving new changes or approving a push.
- Crucial for enforcing guidelines across a team and ensuring a consistent workflow.
A basic comparison between client-side and Server Side hooks
Aspect | Client-Side Hooks (Local Hooks) | Server-Side Hooks |
Scope | Individual developer’s repository | Remote repository |
Location | .git/hooks directory | Host server of the remote repo |
Activation | Local machine events | Remote repository events |
Purpose | Personal development standard | Enforcing team guideline |
Impact | Developer’s workflow | Team collaboration |
How Git Hooks Work?
Imagine Git hooks as your project’s personal assistants. They’re like little programs that Git runs at specific times, kind of like reminders or tasks. Here’s how they work in the simplest terms:
👉You set up a hook: First, you create a special file with a specific name in your Git project folder. This file contains instructions for Git on what to do at a certain time, like before you save your changes (commit) or after you receive new changes (pull).
👉Git listens for triggers: Whenever you do something in Git that matches what a hook is looking for—like trying to save changes (commit) or receive new changes (pull)—Git says, “Hey, I’ve got a job for this hook!”
👉Hook does its job: Git hands over control to the hook, and it does its thing based on what you told it to do. For example, it might run tests on your code, format your files, or send a notification.
👉Result: After the hook finishes its task, Git resumes what it was doing. If the hook was set up to check something before a commit, Git will only let you save your changes (commit) if everything the hook checked passes.
So, Git hooks are like little helpers that jump in at specific moments to help you keep your project organized, checked, and running smoothly!
The Essential Fundamental Of Proper Git Hooks Techniques
Git hooks are like little scripts that automatically run at certain points when you’re working with Git. They’re handy because they let you tweak how Git behaves to suit your project’s needs. Here are the main types of hooks and what they do:
⚙️Pre-commit: These run just before you save your changes. You can use them to check your code for errors or make sure it follows certain style rules.
⚙️Post-commit: These kick in right after you’ve made a commit. They’re useful for things like sending notifications or keeping a log of your changes.
⚙️Pre-push: These fire up before you push your code to a shared repository. They’re great for running tests to make sure your changes won’t break anything.
⚙️Post-push: Finally, these run after you’ve finished pushing your code. They’re handy for tasks like deploying your code to a live website or updating project documentation.
You can find these hooks in the .git/hooks folder of your Git project. There are some example hooks in there that you can use as templates to create your own custom ones. They’re named with a sample- prefix to make it easier for you to get started.
[GitGuardian]
Exploring Git Hooks Parameters And Environment Variables
Git hooks are particularly potent because of their adept handling of dynamic variables, although grasping this concept can prove complex. Let’s delve into both environmental variables
How Parameters Are Sent to Hooks
Hooks can receive certain parameters from Git, allowing them to access contextual information from your main codebase. Git automatically sets these parameters at runtime, so you usually don’t need to define them explicitly. However, it’s important to understand them to create effective hooks.
Here’s a summary of the key points about hook parameters:
- Git hooks use positional variables, where $1 stands for the first parameter, $2 for the second, and so on. These parameters have specific meanings and purposes.
- The order of the parameters follows a specific pattern, determined by the context of the hook event.
- The variable names indicate the general purpose of the parameters. For example, $1 often contains the path to a file, while $2 might be the source of an action.
- If you add a parameter that the hook doesn’t recognize, the script won’t be able to use it. Parameters are specific to each hook and its execution context. To avoid issues, stick to using documented parameters.
Since each Git hook comes with its own parameters, you’ll probably need a guide to figure out what they are for your project. Luckily, there are a few ways to do this.
For instance, you can check out the official Git Hooks documentation, which lists some of the common parameters. But the easiest way is to look at one of the sample Git hooks. These come with instructions on how to write the hook and also include definitions for the parameters.
Environmental Variables
Git hooks can gather arguments from command-line inputs, stdin, and the environment itself while running within a bash shell.
Note: Standard input (stdin) is a programming term that refers to the default device a program uses to get information from the user.
These environment variables allow you to customize how your Git hooks behave and make decisions based on different aspects of your Git workflow. This flexibility enables you to create dynamic and context-aware Git hooks. For instance, you can use them to validate commit messages, control branch access, or trigger custom actions based on the author’s identity.
We suggest referring to the Git documentation and sample hooks for clues on which variables are utilized.
Popular Git Scripting Languages Commonly Used in Git Hooks
Here are some commonly used scripting languages and how they relate to Git hooks:
- Bash (Shell Scripting): Bash is versatile and commonly used for tasks like testing or formatting checks. Git hooks like pre-commit or pre-push are often written in Bash, starting with #!/bin/bash.
- Python: Python is known for its simplicity, making it great for tasks like interacting with APIs or generating reports. Python scripts can be used for Git hooks actions, starting with #!/usr/bin/env python.
- Ruby: Ruby is elegant and readable, making it useful for building and deploying tasks. Ruby scripts can be used for Git hooks, offering an alternative to Bash.
- JavaScript: JavaScript is commonly used with tools like Node.js and in JavaScript-centric projects. While less common, Git hooks can also be written in JavaScript, especially when using Node.js. This is beneficial for projects primarily using JavaScript.
What Is the Scope Of Git Hooks?
Git hooks have specific roles based on where and when they’re used in the version control process, enabling automation and rule enforcement. They operate in two scopes and respond to events like commits and pushes. Let’s explore these scopes:
- Local: These hooks function on a developer’s machine and affect their workflow exclusively, without impacting the central repository or others’ repos.
- Server: These hooks work on the remote repository, influencing the entire team. They respond to events like receiving new changes.
Local hooks impact individuals, while server hooks affect a group of people. However, managing hooks in the .git/hooks directory poses a challenge for teams working on projects using Git hooks.
One solution is to place hooks above the .git directory within the project. This allows users to edit hooks like version-controlled files. To use a hook, create a symlink to it or copy it into .git/hooks when updated.
Basic & Easy Git Hooks Examples
Here are some simple and generic Git hooks examples
Pre-commit Hook:
- Purpose: Run tests or linting before committing changes.
- Example: Check for trailing whitespace before allowing a commit.
#!/bin/bash if git diff –cached –check; then exit 0 else echo “Error: Commit contains trailing whitespace, please fix before committing.” exit 1 fi |
Post-commit Hook:
- Purpose: Send notifications or perform cleanup tasks after committing changes.
- Example: Display a message after each commit.
#!/bin/bash echo “Commit successful! Thank you for contributing.“ |
Pre-push Hook:
- Purpose: Validate code or perform checks before pushing changes.
- Example: Prevent pushing to the main branch directly.
#!/bin/bash protected_branch=”main” current_branch=$(git symbolic-ref HEAD | sed -e ‘s,.*/\(.*\),\1,’) if [ “$current_branch” = “$protected_branch” ]; then echo “Error: You cannot push directly to the main branch.” exit 1fi |
Post-receive Hook:
- Purpose: Trigger deployment or notification actions after receiving changes on the remote repository.
- Example: Automatically deploy changes to a staging server.
#!/bin/bash # Example deployment script git –work-tree=/path/to/staging/folder checkout -f echo “Changes deployed to staging server.” |
These examples cover some common use cases of Git hooks and demonstrate how they can be used to automate tasks, enforce rules, and improve development workflows.
Some Useful GIT Workflow Strategies
Git workflow strategies are crucial for organizing team collaboration, handling changes, and delivering software effectively. Below, we will provide an in-depth look at several commonly used Git workflow strategies:
Single Source Control Workflow
git clone <repository-url> git checkout -b feature-branch # Make changes git add . git commit -m “Implement feature XYZ” git push origin feature-branch |
This is a basic Git workflow, perfect for small teams or projects. There’s one main repository where everyone pushes changes directly. Here’s the gist:
Key Points:
Only one main repository exists, usually with a single main branch like “master” or “main“.
Developers clone the repository, make branches for features or fixes, edit them, and push them straight to the main repository.
Pros:
- Simple and easy to understand.
- Works well for small teams or projects that don’t need a lot of branching.
Cons:
- Not great for big teams or complex projects because limited branching can cause conflicts and coordination problems.
Feature Branching Workflow
git checkout -b feature-branch # Make changes git add . git commit -m “Implement feature XYZ” git push origin feature-branch |
In this Git workflow, each new feature or bug fix gets its own branch. Here’s how it works:
Key Points:
- Developers make feature branches from the main branch.
- They make changes in these branches and then merge them back using pull requests.
- This keeps the main branch clean and organized with separate branches for each feature.
Pros:
- Allows multiple changes to happen at once without messing up the main branch.
- Makes it easy for others to review and collaborate on code through pull requests.
Cons:
- Requires careful management to keep feature branches in sync with the main branch.
Forking GIT Workflow
# Fork repository on GitHub git clone <forked-repository-url> git checkout -b feature-branch # Make changes git add . git commit -m “Implement feature XYZ” git push origin feature-branch |
This Git workflow is popular in open-source projects with many contributors. Here’s how it works:
Key Points:
- Contributors copy the main repository (fork it), make changes in their copy, and then ask for their changes to be included (pull request).
- Contributors manage their copies and send changes to their own branches.
- Project maintainers review and add changes from contributors’ copies into the main repository.
Pros:
- Lets people contribute to a project without directly changing the main one.
- Gives maintainers control and security over what changes get added.
Cons:
- Contributors have to make sure their copies stay up to date with the main one.
Gitflow Workflow
git flow feature start feature-branch # Make changes git flow feature finish feature-branch |
This Git workflow, called Gitflow, is great for projects with regular releases and lots of different environments. Here’s how it works:
Key Points:
- It has special branches for different things like making new features, releasing updates, and fixing bugs quickly.
- It uses branches like “develop” for ongoing work and “master” for finished updates.
- When new features are done, they get added to both the main branches.
Pros:
- Gives a clear plan for keeping track of versions, especially for bigger teams and complicated projects.
- Sets clear roles for who does what when releasing updates.
Cons:
- Can get pretty complicated with lots of branches and keeping track of releases.
Best Git Hooks Practices for Best Efficiency
Yet, for effective codebase management, organization, and maintainability, developers must adhere to certain best practices. Here, we’ll explore several recommended practices for utilizing Git.
Proper Validation & Error Handling
It’s important to focus on making sure your Git hooks work reliably and safely. This means paying attention to how you handle errors and validate inputs.
For instance, you should always check any information your hook scripts get. But there’s more to it than that. You could also make sure that your repository is set up correctly for the hook to work right. For example, in a pre-commit hook, you’d want to double-check that you’ve added all the files you need before you make a commit.
Preventing Accidental Harm
Accidents can happen, so it’s vital to set up your Git hooks to stop these accidental destructive actions. This helps protect against losing or damaging data. Hooks act like safety nets by asking users before they do something that could cause harm.
Two useful hooks for this are pre-receive and pre-commit:
👉Pre-receive hooks work on the server and check things before accepting changes from users. They can look for risky actions like force pushes or deleting branches and ask for confirmation before accepting them.
👉Pre-commit hooks run on your computer before you finalize a commit. They don’t stop bad things on the server, but they can catch mistakes before you send changes. They check things like commit messages for risky commands and warn you if they find any.
But no matter what you do, it’s important that your practices are safe, effective, and right for what you need. This means reviewing and testing them carefully.
Assessing And Validating Git Hooks
Checking and testing hooks is really important to make sure they work right and fit with how you work on projects. Peer reviews, clear instructions, lots of comments, and other stuff can help make sure hooks are good to go.
When you test, it’s best to do it by itself with lots of different kinds of examples. You might also want to set up automatic testing to catch any problems as soon as they happen.
Frequent Commit of Git
A good rule for Git is to save your work often by making commits. It’s best to make lots of small commits, each one showing a single change you made. This way, it’s simple to see what you did and go back to an earlier version if needed. Also, it’s important to write clear messages when you commit, so others know what you changed and why.
Picking Clear Branch Names
Another good idea is to give branches names that make sense. Branch names should tell you what the branch is for. This helps everyone understand what’s going on and prevents mix-ups. It’s also a good idea to use feature branches. These are branches made just for working on a new feature or fixing a bug. They keep your changes separate from the main code until they’re ready to be added in.
Keep Your Git Repository Clean
Keeping your repository neat and tidy is important. You should get rid of any files or folders you don’t need. This makes the repository smaller and simpler to use. Also, use Gitignore files to tell Git to ignore certain files, like temporary ones or things you build, so they don’t clutter up your project.
Use Proper Git Tags
Git tags are like stickers you put on certain commits to show they’re important. They can say things like “version 1.0” or “release 2.0”. Developers use tags to highlight big moments, like when they’re ready to share their work or when they fix a big problem. Tags help keep track of changes and make it easy to see what’s happened over time.
Properly Test Your Git Hooks
Before you deploy hooks for use or share them with your team, it’s crucial to put them through thorough testing. This ensures they function as expected and won’t cause any problems in your workflow. Testing hooks involves running them in various scenarios to verify their reliability and effectiveness. Additionally, it’s essential to check for any unintended consequences or conflicts with existing processes. By testing hooks before implementation, you can mitigate potential issues and ensure a smoother integration into your development workflow.
Engineering Sturdy Git Hooks
It’s important to ensure that your hooks can handle various situations smoothly and reliably. This means making them robust enough to deal with different scenarios effectively. Additionally, it’s crucial to make them portable by considering compatibility across different platforms. Avoid relying on specific paths or dependencies that may not be available everywhere. By doing so, you can ensure that your hooks are adaptable and functional across different environments, enhancing their usability and effectiveness in your workflow.
Clone a Website From Git Repository with xCloud
With xCloud, cloning a website from a Git Repository is incredibly simple, with no complexity involved. The migration process is remarkably fast, ensuring a swift transition without making any changes to the code in your Git repository. Migrating a website from a Git repository with xCloud entails several straightforward steps. This guide can assist you in comprehending how to execute this migration effortlessly.
Optimize Web Development with the Best Git Hooks Practices
In conclusion, Git hooks serve as powerful tools in the developer’s arsenal, offering a wide array of functionalities to streamline workflows, enhance collaboration, and maintain code quality. By implementing best practices such as thorough testing, meaningful branch naming, and robust coding, developers can leverage Git hooks to automate tasks, enforce standards, and optimize their development processes.
Whether it’s ensuring code quality with pre-commit hooks, automating deployment processes with post-receive hooks, or facilitating collaboration with pre-push hooks, Git hooks provide endless possibilities for improving efficiency and productivity in software development.
If you have found this blog helpful, feel free to subscribe to our blogs for valuable tutorials, guides, knowledge, and tips on web hosting and server management. You can also join our Facebook community to share insights and engage in discussions.